一、SpringBoot简介
传统Spring开发缺点:
1、导入依赖繁琐
2、项目配置繁琐
Spring Boot是全新框架(更像是一个工具, 脚手架),是Spring提供的一个子项目, 用于快速构建Spring应用程序。
随着Spring 3.0的发布,Spring 团队逐渐开始摆脱XML配置文件,并且在开发过程中大量使用“约定优先配置”(convention over configuration)的思想来摆脱Spring框架中各类繁复纷杂的配置。
SpringBoot的特性:
- 起步依赖 jar包的管理 starter
- 自动配置 SpringBoot做了很多默认的配置
- 内置tomcat
二、创建SpringBoot项目
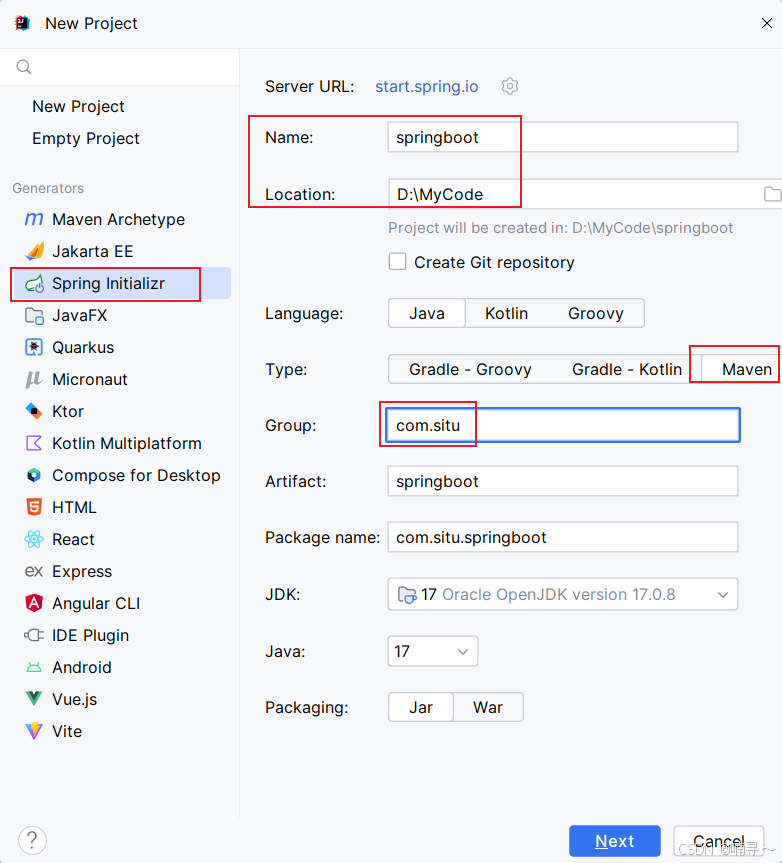
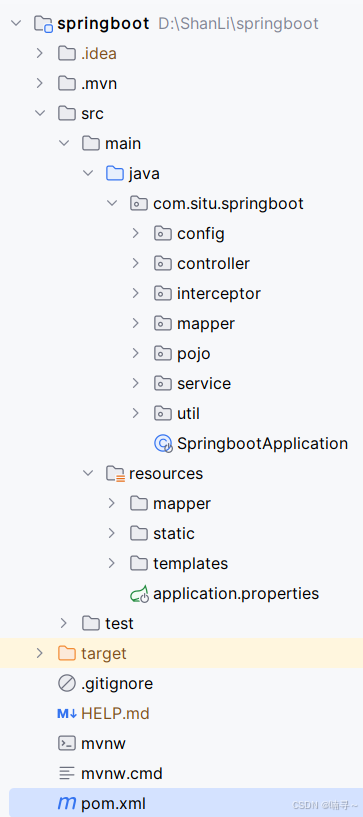
1、pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><parent><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-parent</artifactId><version>3.2.5</version><relativePath/> <!-- lookup parent from repository --></parent><groupId>com.situ</groupId><artifactId>springboot</artifactId><version>0.0.1-SNAPSHOT</version><name>springboot</name><description>springboot</description><properties><java.version>17</java.version></properties><dependencies><!--Spring相关依赖--><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter</artifactId></dependency><!--SpringMVC相关依赖--><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-web</artifactId></dependency><!-- mysql驱动 --><dependency><groupId>mysql</groupId><artifactId>mysql-connector-java</artifactId><version>8.0.31</version></dependency><!-- mybatis --><dependency><groupId>org.mybatis.spring.boot</groupId><artifactId>mybatis-spring-boot-starter</artifactId>f<version>3.0.3</version></dependency><!--thymeleaf相关依赖--><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-thymeleaf</artifactId></dependency><!--lombok帮助我们生成实体类的构造方法、get、set、toString--><dependency><groupId>org.projectlombok</groupId><artifactId>lombok</artifactId><version>1.18.14</version><scope>provided</scope></dependency><!-- 分页插件 --><dependency><groupId>com.github.pagehelper</groupId><artifactId>pagehelper-spring-boot-starter</artifactId><version>1.4.1</version></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-test</artifactId><scope>test</scope></dependency></dependencies><build><plugins><plugin><groupId>org.springframework.boot</groupId><artifactId>spring-boot-maven-plugin</artifactId></plugin></plugins></build></project>
2、SpringbootApplication启动类
//SpringBoot项目启动类
@SpringBootApplication
@MapperScan("com.situ.springboot.mapper")
public class SpringbootApplication {public static void main(String[] args) {SpringApplication.run(SpringbootApplication.class, args);}
}
@Controller
@RequestMapping("/admin")
public class AdminController {@RequestMapping("/selectAll")@ResponseBodypublic List<Admin> selectAll() {System.out.println("AdminController.selectAll");List<Admin> list = new ArrayList<>();Admin admin1 = new Admin();admin1.setId(1);admin1.setName("zhangsan");Admin admin2 = new Admin();admin2.setId(2);admin2.setName("list");Admin admin3 = new Admin();admin3.setId(3);admin3.setName("wangwu");list.add(admin1);list.add(admin2);list.add(admin3);return list;}
}
3、application.properties
server.port=8080#DB Configuration
spring.datasource.driverClassName=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/blog?useSSL=false&useUnicode=true&characterEncoding=utf8&serverTimezone=GMT%2b8&zeroDateTimeBehavior=CONVERT_TO_NULL
spring.datasource.username=root
spring.datasource.password=1234spring.mvc.static-path-pattern=/static/**#Spring MyBatis
mybatis.type-aliases-package=com.situ.springboot.pojo
mybatis.mapper-locations=classpath:mapper/*Mapper.xml
mybatis.configuration.map-underscore-to-camel-case=true
#log
mybatis.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
4、AdminMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapperPUBLIC "-//mybatis.org//DTD Mapper 3.0//EN""http://mybatis.org/dtd/mybatis-3-mapper.dtd"><mapper namespace="com.situ.springboot.mapper.AdminMapper"><select id="selectAll" resultType="Admin">SELECT id,name,password,nick_name,role,image FROM admin</select>
</mapper>
三、Thymeleaf
<!--thymeleaf相关依赖-->
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
application.properties:
spring.mvc.static-path-pattern=/static/**
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org/">
<head><title>主页 </title><link rel="stylesheet" type="text/css" th:href="@{/static/layui/css/layui.css}"/><script th:src="@{/static/layui/layui.js}" type="text/javascript" charset="utf-8"></script>
</head>
@Controller
@RequestMapping("/page")
public class PageController {/*** /page/user/add /page/login** @return*/@RequestMapping("/**")public String path(HttpServletRequest request) {String requestURI = request.getRequestURI();System.out.println("requestURI: " + requestURI);String[] paths = requestURI.split("/");//["","page","user","list"]//["","page","login"]if (paths.length == 4) {return paths[2] + "_" + paths[3];} else if (paths.length == 3) {return paths[2];} else {return "index";}}
}
四、登录拦截器
//拦截器的作用:浏览器访问服务器的请求,都要首先经过拦截器
public class LoginInterceptor implements HandlerInterceptor {@Overridepublic boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {////1.判断用户有没有登录//2.如果登录了,就放行,可以访问后台的资源//3.如果没有登录,跳转到登录界面HttpSession session = request.getSession();Admin admin = (Admin) session.getAttribute("admin");if (admin == null) {//没有登录,跳转到登录界面response.sendRedirect("/page/login");return false;}//已经登录,放行return true;}
}
拦截器配置
WebMvcConfigurer提供了配置SpringMVC底层的所有组件入口
// @Component <bean>
// @Controller @Service @Repository
// 这四个注解的作用是一样的,下面三个不一样主要是为了区分不同层// @Configuration用于定义配置类,可以替换xml配置文件,
// 加了这个注解的类的内部包含一个或多个被@Bean注解的方法
@Configuration
public class WebConfig implements WebMvcConfigurer {/* <bean name="admin" class="com.situ.springboot.pojo.Admin"/>*/@Beanpublic Admin createAdmin() {return new Admin();}//配置虚拟路径@Overridepublic void addResourceHandlers(ResourceHandlerRegistry registry) {registry.addResourceHandler("/pic/**").addResourceLocations("file:/D:/mypic/");WebMvcConfigurer.super.addResourceHandlers(registry);}/*<!-- 配置拦截器 --><mvc:interceptors><mvc:interceptor><mvc:mapping path="/**"/><mvc:exclude-mapping path=""/><bean class="com.situ.mvc.interceptor.MyInterceptor1"></bean></mvc:interceptor></mvc:interceptors>*/// 这个方法用来注册拦截器,我们写的拦截器需要在这里配置才能生效@Overridepublic void addInterceptors(InterceptorRegistry registry) {//把登录的拦截器配置上才能起作用// addPathPatterns("/**") 拦截器拦截所有的请求,静态资源也拦截了,需要放行// excludePathPatterns 代表哪些请求不需要拦截registry.addInterceptor(new LoginInterceptor()).addPathPatterns("/**").excludePathPatterns("/page/login", "/admin/login", "/admin/logout", "/static/**");}
}