In this article, we'll see how we can remove a CSS property from a certain element using JavaScript? We can remove only those properties that we assign ourselves and the pre-default ones cannot be removed by this method.
在本文中,我们将看到如何使用JavaScript从某个元素中删除CSS属性? 我们只能删除分配给我们的属性,而默认属性不能被此方法删除。
HTML:
HTML:
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Removing CSS property</title>
<!-- Compiled and minified CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css">
<!-- Compiled and minified JavaScript -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script>
</head>
<style>
body {
background: silver;
}
h2 {
background: wheat;
padding: 10px;
}
.btn {
background: tomato;
}
.text {
font-weight: 500;
}
</style>
<body>
<div class="container">
<h2>Let's remove some css!</h2>
<div class="container">
<button class="btn">Just a random button!</button>
<p class="text">Lorem ipsum, dolor sit amet consectetur adipisicing elit. Tempora quis asperiores dicta quos laborum laboriosam perferendis ab veniam odit saepe, obcaecati officiis iure iste voluptates at quod commodi cupiditate voluptas!</p>
</div>
</div>
</body>
<script>
</script>
</html>
Output
输出量
We can call on a certain DOM selector's style property and use the remove property method to remove that particular CSS property. First, let's get a reference to all the DOM elements.
我们可以调用某个DOM选择器的style属性,并使用remove属性方法删除该特定CSS属性。 首先,让我们参考所有DOM元素。
<script>
const heading = document.querySelector('h2');
const button = document.querySelector('.btn');
const para = document.querySelector('.text');
</script>
Now let's try to remove the background property from our heading,
现在,让我们尝试从标题中删除background属性,
heading.style.removeProperty("background");
Oops! That didn't quite work, did it? There's a simple explanation. We are loading our scripts when our whole page loads and the styles are written inside our stylesheet. Even if we remove a certain property using JavaScript, it wouldn't make any difference since that DOM element is also hooked to a stylesheet and we aren't removing any kind of CSS that we have written. Let's refactor our code a little bit now, let's clear out styles and apply those styles using JavaScript,
糟糕! 那不是很有效,对吗? 有一个简单的解释。 当整个页面加载并且样式写在样式表中时,我们正在加载脚本。 即使我们使用JavaScript删除某个属性,也不会有任何区别,因为该DOM元素也被挂钩到样式表,并且我们也不会删除我们编写的任何CSS。 现在,让我们重构一下代码,清除样式并使用JavaScript应用这些样式,
<style>
/* body{
background: silver;
}
h2{
background: wheat;
padding: 10px;
}
.btn{
background: tomato;
}
.text{
font-weight: 500;
} */
</style>
Output
输出量
As you can see now all our styles are removed. Let's add them back in our JavaScript,
如您所见,我们的所有样式均已删除。 让我们将它们重新添加到JavaScript中,
<script>
const heading = document.querySelector('h2');
const button = document.querySelector('.btn');
const para = document.querySelector('.text');
heading.style.background = "wheat";
heading.style.padding = "10px";
document.querySelector('body').style.background = "silver";
button.style.background = "tomato";
para.style.fontWeight = "500";
</script>
Output
输出量
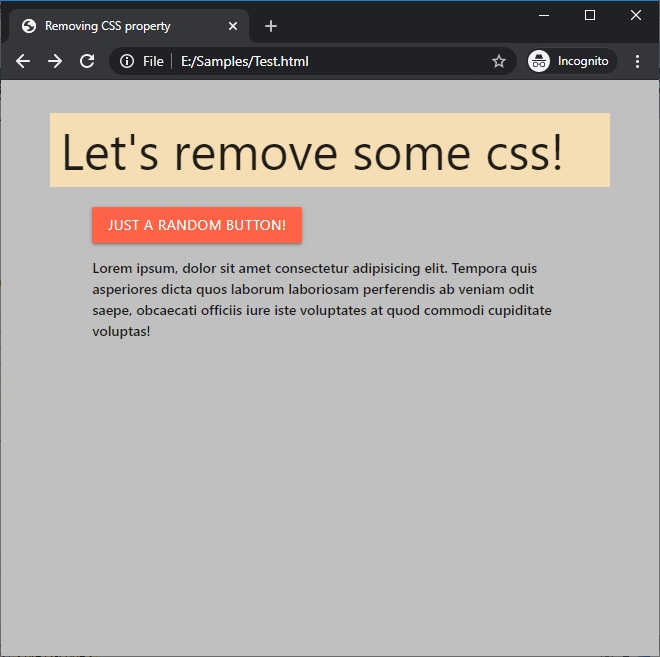
And we have our styles back! Great. Now let's try removing them using our JavaScript,
我们的风格又回来了! 大。 现在,让我们尝试使用我们JavaScript删除它们,
heading.style.removeProperty("background");
Output
输出量
Our heading no longer has a wheat background! Great. Let's remove all the CSS properties inside a function and see if we get back the same unstyled page.
我们的标题不再具有小麦背景! 大。 让我们删除函数内的所有CSS属性,看看是否返回相同的未样式化页面。
function removeProperties() {
document.querySelector('body').style.removeProperty("background");
heading.style.removeProperty("background");
heading.style.removeProperty("padding");
button.style.removeProperty("background");
para.style.removeProperty("fontWeight");
}
Output
输出量
Everything should remain the same as we have not yet called or invoked our function so let's do it now inside our console,
一切都应该保持不变,因为我们尚未调用或调用函数,因此现在让我们在控制台中进行操作,
removeProperties();
Output
输出量
Voila! We have successfully removed all our CSS properties using JavaScript! Can you check for yourself is this method works for inline styles?
瞧! 我们已经使用JavaScript成功删除了所有CSS属性 ! 您可以自己检查一下这种方法是否适用于内联样式?
翻译自: https://www.includehelp.com/code-snippets/how-to-remove-css-property-using-javascript.aspx