文章目录
- 背景
- 一般的实现
- 使用spring-boot-dependencies 更优雅的实现.
背景
有时候构建一个多模块maven项目其中某一个模块是web-service需要使用spring boot,其他模块跟spring boot 完全无关,本文总结一下在这个场景下maven项目最佳构建方式.
一般的实现
网上应该也看到过很多人写的文章,思路非常简单,即是将整个项目作为spring-boot-starter-parent 的子项目. 这样整个项目里面所有模块都可以在dependencies里面添加上有parent内指定版本的spring boot 相关的起步依赖包. 而不需要使用spring的模块可以完全不用管这个parent pom.
比如要构建如下含有三个模块的maven project:
module name | description |
---|---|
core | 存放一些公共的类的模块,可被其他模块使用 |
web-service | spring boot service 对外提供rest api |
spark-job | spark 项目 |
project的pom.xml如下:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><parent><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-parent</artifactId><version>2.7.18</version></parent><groupId>org.mytest</groupId><artifactId>demo1</artifactId><version>1.0-SNAPSHOT</version><packaging>pom</packaging><modules><module>web-service</module><module>spark-job</module><module>core</module></modules><properties><maven.compiler.source>11</maven.compiler.source><maven.compiler.target>11</maven.compiler.target><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding></properties></project>
core 模块的pom.xml如下:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><parent><groupId>org.mytest</groupId><artifactId>demo1</artifactId><version>1.0-SNAPSHOT</version></parent><artifactId>core</artifactId><properties><maven.compiler.source>11</maven.compiler.source><maven.compiler.target>11</maven.compiler.target><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding></properties></project>
web-service的pom如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><parent><groupId>org.mytest</groupId><artifactId>demo1</artifactId><version>1.0-SNAPSHOT</version></parent><artifactId>web-service</artifactId><properties><maven.compiler.source>11</maven.compiler.source><maven.compiler.target>11</maven.compiler.target><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding></properties><dependencies><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-web</artifactId></dependency></dependencies><build><plugins><plugin><groupId>org.springframework.boot</groupId><artifactId>spring-boot-maven-plugin</artifactId></plugin></plugins></build>
</project>
spark-job的pom如下(大致的pom不讨论细节)
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><parent><groupId>org.mytest</groupId><artifactId>demo1</artifactId><version>1.0-SNAPSHOT</version></parent><artifactId>spark-job</artifactId><properties><maven.compiler.source>11</maven.compiler.source><maven.compiler.target>11</maven.compiler.target><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding></properties></project>
这样构建的项目由于使用spring-boot-starter-parent 作为parent pom所以整个项目其实是下图的结构:
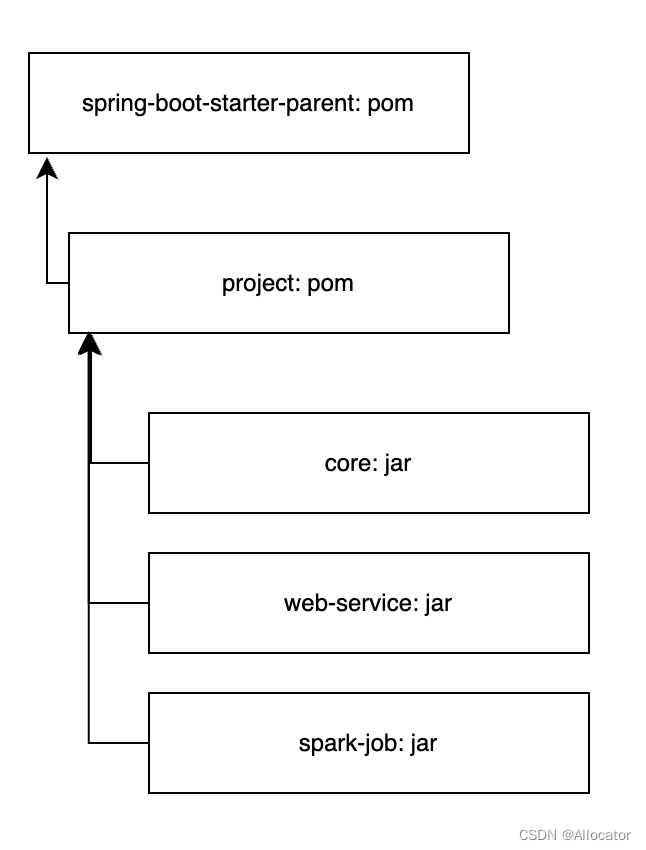
这个结构看起来不是那么舒服,比如这里买的spark-job模块,跟spring完全没关, 不应该让spring-boot-started-parent作为其parent.那么有没有什么方法能够不使用spring-boot-starter-parent作为整个项目的parent也能完全解决spring boot依赖的问题呢?答案是有的.
使用spring-boot-dependencies 更优雅的实现.
spring 本身提供了这样一个pom 来管理所有依赖: https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-dependencies
且官方也给了怎么使用这个pom的方法
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-dependencies</artifactId><version>2.7.18</version><type>pom</type><scope>import</scope>
</dependency>
有了这个pom引入到项目里面,就可以不用将spring-boot-starter-parent作为整个项目的parent.
改造一下项目的pom
project pom.xml 如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><groupId>org.mytest</groupId><artifactId>demo2</artifactId><version>${revision}</version><packaging>pom</packaging><modules><module>core</module><module>web-service</module><module>spark-job</module></modules><properties><maven.compiler.source>11</maven.compiler.source><maven.compiler.target>11</maven.compiler.target><!--revision统一版本号--><revision>1.0</revision><spring.boot.version>2.7.18</spring.boot.version><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding></properties><dependencyManagement><dependencies><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-dependencies</artifactId><version>${spring.boot.version}</version><type>pom</type><scope>import</scope></dependency><!--core模块依赖声明--><dependency><groupId>org.mytest</groupId><artifactId>core</artifactId><version>${project.version}</version></dependency></dependencies></dependencyManagement></project>
core 模块 pom.xml 如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><parent><groupId>org.mytest</groupId><artifactId>demo2</artifactId><version>${revision}</version></parent><artifactId>core</artifactId><properties><maven.compiler.source>11</maven.compiler.source><maven.compiler.target>11</maven.compiler.target><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding></properties></project>
web-service的pom.xml如下:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><parent><groupId>org.mytest</groupId><artifactId>demo2</artifactId><version>${revision}</version></parent><artifactId>web-service</artifactId><properties><maven.compiler.source>11</maven.compiler.source><maven.compiler.target>11</maven.compiler.target><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding></properties><dependencies><!--web-service中引入core模块--><dependency><groupId>org.mytest</groupId><artifactId>core</artifactId><version>${project.version}</version></dependency><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-web</artifactId></dependency></dependencies></project>
spark-job的pom.xml(大致的pom不讨论细节)如下
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"><modelVersion>4.0.0</modelVersion><parent><groupId>org.mytest</groupId><artifactId>demo2</artifactId><version>${revision}</version></parent><artifactId>spark-job</artifactId><properties><maven.compiler.source>11</maven.compiler.source><maven.compiler.target>11</maven.compiler.target><project.build.sourceEncoding>UTF-8</project.build.sourceEncoding></properties></project>
改进之后项目就不存在以spring-boot-starter-parent作为整个项目的parent实现spring boot依赖包的管理. 项目结构如下:
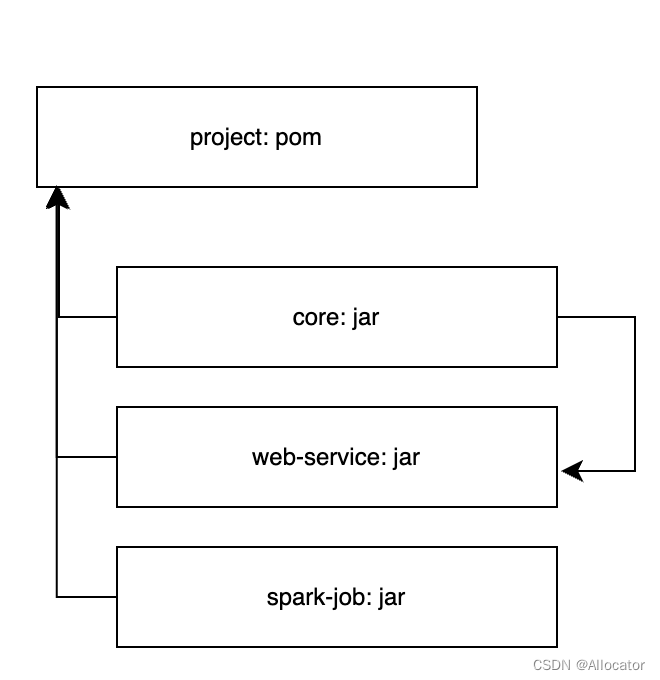