Our-task介绍
本篇博客是我github上our-task:一个完整的清单管理系统的配套教程文档,这是SpringBoot+Vue开发的前后端分离清单管理工具,仿滴答清单。目前已部署在阿里云ECS上,可进行在线预览,随意使用(附详细教程),大家感兴趣的话,欢迎给个star!
阿里云预览地址
Redis的安装与配置
Windows下redis的安装与配置
SpringBoot整合Redis
添加项目依赖
<dependencies><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-web</artifactId></dependency>
<dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-test</artifactId><scope>test</scope></dependency><!--redis依赖配置--><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-data-redis</artifactId></dependency>
</dependencies>
yml文件配置
yml文件中主要是关于Redis的配置,其中键的设置格式为:数据库名:表名:id,更多Redis了解,请大家参考这篇文章。
Redis各种键的典型使用场景,不清楚的都来看一下
# DataSource Config
spring:redis:# Redis服务器地址host: localhost# Redis数据库索引(默认为0)database: 0# Redis服务器连接端口port: 6379# Redis服务器连接密码(默认为空)password:jedis:pool:# 连接池最大连接数(使用负值表示没有限制)max-active: 8# 连接池最大阻塞等待时间(使用负值表示没有限制)max-wait: -1ms# 连接池中的最大空闲连接max-idle: 8# 连接池中的最小空闲连接min-idle: 0# 连接超时时间(毫秒)timeout: 3000ms
# 自定义redis key
redis:database: "study"key:user: "user"expire:common: 86400 # 24小时
实体类
新建entity包,在该包下新建User类,用作该Demo的操作实体。考虑到一些小白可能还不了解Lombok,我就直接使用Setter/Getter。
package com.example.demo.entity;
/*** @program: SpringBoot-Redis-Demo* @description: 用户类* @author: water76016* @create: 2020-12-29 09:22**/
public class User {Integer id;String username;String password;
public User(Integer id, String username, String password) {this.id = id;this.username = username;this.password = password;}
@Overridepublic String toString() {return "User{" +"username='" + username + ''' +", password='" + password + ''' +'}';}
public Integer getId() {return id;}
public void setId(Integer id) {this.id = id;}
public String getUsername() {return username;}
public void setUsername(String username) {this.username = username;}
public String getPassword() {return password;}
public void setPassword(String password) {this.password = password;}
}
Service服务接口
服务接口一共有两个:一个是UserService,一个是RedisService。UserService提供了针对User实体的服务,RedisService提供操作Reids的服务接口。
package com.example.demo.service;
import com.example.demo.entity.User;
public interface UserService {/*** 对用户打招呼,返回user的toString()方法* @param user* @return*/public String helloUser(User user);
}
package com.example.demo.service;
import java.util.List;
import java.util.Map;
import java.util.Set;
/*** @program: our-task* @description: redis操作服务类* @author: water76016* @create: 2020-09-24 16:45**/
public interface RedisService {
/*** 保存属性** @param key the key* @param value the value* @param time the time*/void set(String key, Object value, long time);
/*** 保存属性** @param key 键* @param value 值*/void set(String key, Object value);
/*** 获取属性** @param key the key* @return the object*/Object get(String key);
/*** 删除属性** @param key the key* @return the boolean*/Boolean del(String key);
/*** 批量删除属性** @param keys the keys* @return the long*/Long del(List<String> keys);
/*** 设置过期时间** @param key the key* @param time the time* @return the boolean*/Boolean expire(String key, long time);
/*** 获取过期时间** @param key the key* @return the expire*/Long getExpire(String key);
/*** 判断是否有该属性** @param key the key* @return the boolean*/Boolean hasKey(String key);
/*** 按delta递增** @param key the key* @param delta the delta* @return the long*/Long incr(String key, long delta);
/*** 按delta递减** @param key the key* @param delta the delta* @return the long*/Long decr(String key, long delta);
/*** 获取Hash结构中的属性** @param key the key* @param hashKey the hash key* @return the object*/Object hGet(String key, String hashKey);
/*** 向Hash结构中放入一个属性** @param key the key* @param hashKey the hash key* @param value the value* @param time the time* @return the boolean*/Boolean hSet(String key, String hashKey, Object value, long time);
/*** 向Hash结构中放入一个属性** @param key the key* @param hashKey the hash key* @param value the value*/void hSet(String key, String hashKey, Object value);
/*** 直接获取整个Hash结构** @param key the key* @return the map*/Map<Object, Object> hGetAll(String key);
/*** 直接设置整个Hash结构** @param key the key* @param map the map* @param time the time* @return the boolean*/Boolean hSetAll(String key, Map<String, Object> map, long time);
/*** 直接设置整个Hash结构** @param key the key* @param map the map*/void hSetAll(String key, Map<String, Object> map);
/*** 删除Hash结构中的属性** @param key the key* @param hashKey the hash key*/void hDel(String key, Object... hashKey);
/*** 判断Hash结构中是否有该属性** @param key the key* @param hashKey the hash key* @return the boolean*/Boolean hHasKey(String key, String hashKey);
/*** Hash结构中属性递增** @param key the key* @param hashKey the hash key* @param delta the delta* @return the long*/Long hIncr(String key, String hashKey, Long delta);
/*** Hash结构中属性递减** @param key the key* @param hashKey the hash key* @param delta the delta* @return the long*/Long hDecr(String key, String hashKey, Long delta);
/*** 获取Set结构** @param key the key* @return the set*/Set<Object> sMembers(String key);
/*** 向Set结构中添加属性** @param key the key* @param values the values* @return the long*/Long sAdd(String key, Object... values);
/*** 向Set结构中添加属性** @param key the key* @param time the time* @param values the values* @return the long*/Long sAdd(String key, long time, Object... values);
/*** 是否为Set中的属性** @param key the key* @param value the value* @return the boolean*/Boolean sIsMember(String key, Object value);
/*** 获取Set结构的长度** @param key the key* @return the long*/Long sSize(String key);
/*** 删除Set结构中的属性** @param key the key* @param values the values* @return the long*/Long sRemove(String key, Object... values);
/*** 获取List结构中的属性** @param key the key* @param start the start* @param end the end* @return the list*/List<Object> lRange(String key, long start, long end);
/*** 获取List结构的长度** @param key the key* @return the long*/Long lSize(String key);
/*** 根据索引获取List中的属性** @param key the key* @param index the index* @return the object*/Object lIndex(String key, long index);
/*** 向List结构中添加属性** @param key the key* @param value the value* @return the long*/Long lPush(String key, Object value);
/*** 向List结构中添加属性** @param key the key* @param value the value* @param time the time* @return the long*/Long lPush(String key, Object value, long time);
/*** 向List结构中批量添加属性** @param key the key* @param values the values* @return the long*/Long lPushAll(String key, Object... values);
/*** 向List结构中批量添加属性** @param key the key* @param time the time* @param values the values* @return the long*/Long lPushAll(String key, Long time, Object... values);
/*** 从List结构中移除属性** @param key the key* @param count the count* @param value the value* @return the long*/Long lRemove(String key, long count, Object value);
}
Service实现类
在UserService中,我们把用户的信息存储在Redis中。调用了RedisService的方法,同时给该键设置过期时间。大家在操作的时候,直接使用RedisService中的方法就可以了,这些方法还是挺全的。
package com.example.demo.service.impl;
import com.example.demo.entity.User;
import com.example.demo.service.RedisService;
import com.example.demo.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
/*** @program: SpringBoot-Redis-Demo* @description: 用户服务实现类* @author: water76016* @create: 2020-12-29 09:24**/
@Service
public class UserServiceImpl implements UserService {@AutowiredRedisService redisService;
@Value("${redis.database}")private String redisDatabase;@Value("${redis.key.user}")private String redisUserKey;@Value("${redis.expire.common}")private long expire;/*** 对用户打招呼,返回user的toString()方法** @param user* @return*/@Overridepublic String helloUser(User user) {//设置键,键的格式为:数据库名:user:用户idString key = redisDatabase + ":" + redisUserKey + ":" + user.getId();//把用户的toString,存在这个键里面redisService.set(key, user.toString());//设置键的过期时间redisService.expire(key, expire);return user.toString();}
}
package com.example.demo.service.impl;
import com.example.demo.service.RedisService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.RedisSerializer;
import org.springframework.data.redis.serializer.StringRedisSerializer;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.TimeUnit;
/*** @program: our-task* @description: redis操作服务接口实现类* @author: water76016* @create: 2020-09-24 16:45**/
@Service
public class RedisServiceImpl implements RedisService {/*** 由于没有指定具体类型<String, Object>,用Resource注入才有效* */@Resourceprivate RedisTemplate redisTemplate;
@Autowired(required = false)public void setRedisTemplate(RedisTemplate redisTemplate) {RedisSerializer stringSerializer = new StringRedisSerializer();redisTemplate.setKeySerializer(stringSerializer);redisTemplate.setValueSerializer(stringSerializer);redisTemplate.setHashKeySerializer(stringSerializer);redisTemplate.setHashValueSerializer(stringSerializer);this.redisTemplate = redisTemplate;}@Overridepublic void set(String key, Object value, long time) {redisTemplate.opsForValue().set(key, value, time, TimeUnit.SECONDS);}
@Overridepublic void set(String key, Object value) {
redisTemplate.opsForValue().set(key, value);}
@Overridepublic Object get(String key) {return redisTemplate.opsForValue().get(key);}
@Overridepublic Boolean del(String key) {return redisTemplate.delete(key);}
@Overridepublic Long del(List<String> keys) {return redisTemplate.delete(keys);}
@Overridepublic Boolean expire(String key, long time) {return redisTemplate.expire(key, time, TimeUnit.SECONDS);}
@Overridepublic Long getExpire(String key) {return redisTemplate.getExpire(key, TimeUnit.SECONDS);}
@Overridepublic Boolean hasKey(String key) {return redisTemplate.hasKey(key);}
@Overridepublic Long incr(String key, long delta) {return redisTemplate.opsForValue().increment(key, delta);}
@Overridepublic Long decr(String key, long delta) {return redisTemplate.opsForValue().increment(key, -delta);}
@Overridepublic Object hGet(String key, String hashKey) {return redisTemplate.opsForHash().get(key, hashKey);}
@Overridepublic Boolean hSet(String key, String hashKey, Object value, long time) {redisTemplate.opsForHash().put(key, hashKey, value);return expire(key, time);}
@Overridepublic void hSet(String key, String hashKey, Object value) {redisTemplate.opsForHash().put(key, hashKey, value);}
@Overridepublic Map<Object, Object> hGetAll(String key) {return redisTemplate.opsForHash().entries(key);}
@Overridepublic Boolean hSetAll(String key, Map<String, Object> map, long time) {redisTemplate.opsForHash().putAll(key, map);return expire(key, time);}
@Overridepublic void hSetAll(String key, Map<String, Object> map) {redisTemplate.opsForHash().putAll(key, map);}
@Overridepublic void hDel(String key, Object... hashKey) {redisTemplate.opsForHash().delete(key, hashKey);}
@Overridepublic Boolean hHasKey(String key, String hashKey) {return redisTemplate.opsForHash().hasKey(key, hashKey);}
@Overridepublic Long hIncr(String key, String hashKey, Long delta) {return redisTemplate.opsForHash().increment(key, hashKey, delta);}
@Overridepublic Long hDecr(String key, String hashKey, Long delta) {return redisTemplate.opsForHash().increment(key, hashKey, -delta);}
@Overridepublic Set<Object> sMembers(String key) {return redisTemplate.opsForSet().members(key);}
@Overridepublic Long sAdd(String key, Object... values) {return redisTemplate.opsForSet().add(key, values);}
@Overridepublic Long sAdd(String key, long time, Object... values) {Long count = redisTemplate.opsForSet().add(key, values);expire(key, time);return count;}
@Overridepublic Boolean sIsMember(String key, Object value) {return redisTemplate.opsForSet().isMember(key, value);}
@Overridepublic Long sSize(String key) {return redisTemplate.opsForSet().size(key);}
@Overridepublic Long sRemove(String key, Object... values) {return redisTemplate.opsForSet().remove(key, values);}
@Overridepublic List<Object> lRange(String key, long start, long end) {return redisTemplate.opsForList().range(key, start, end);}
@Overridepublic Long lSize(String key) {return redisTemplate.opsForList().size(key);}
@Overridepublic Object lIndex(String key, long index) {return redisTemplate.opsForList().index(key, index);}
@Overridepublic Long lPush(String key, Object value) {return redisTemplate.opsForList().rightPush(key, value);}
@Overridepublic Long lPush(String key, Object value, long time) {Long index = redisTemplate.opsForList().rightPush(key, value);expire(key, time);return index;}
@Overridepublic Long lPushAll(String key, Object... values) {return redisTemplate.opsForList().rightPushAll(key, values);}
@Overridepublic Long lPushAll(String key, Long time, Object... values) {Long count = redisTemplate.opsForList().rightPushAll(key, values);expire(key, time);return count;}
@Overridepublic Long lRemove(String key, long count, Object value) {return redisTemplate.opsForList().remove(key, count, value);}
}
UserController控制器
接下来,我们新建一个controller包,在该包下新建UserController控制类。
package com.example.demo.controller;
import com.example.demo.entity.User;
import com.example.demo.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
/*** @program: SpringBoot-Redis-Demo* @description:* @author: water76016* @create: 2020-12-29 09:27**/
@RestController
@RequestMapping("/user")
public class UserController {@AutowiredUserService userService;
@PostMapping("/helloUser")public String helloUser(@RequestBody User user){return userService.helloUser(user);}
}
结果测试
最后,我们启动SpringBoot程序,程序运行在8080端口,在postman中进行测试。大家按照我在postman中的输入,就会有相应的输出了。
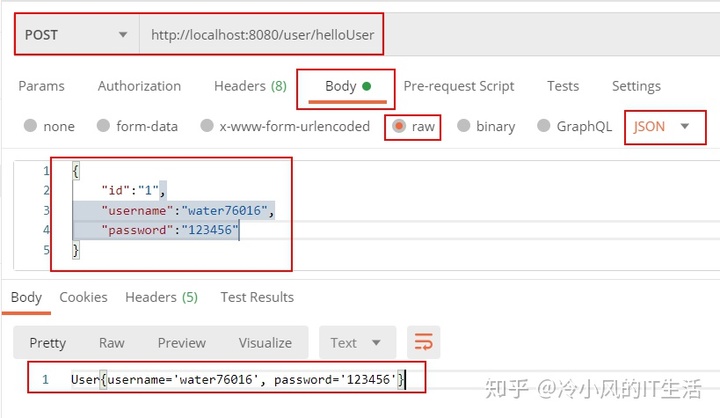
另外,也来看看Redis中是否有相应的缓存结果。

Demo地址
写了这么多,还是担心大家使用的时候有问题,所以我把该Demo放在了Github上,大家自行下载就可以了。
SpringBoot-Redis-Demo地址