期望DP的一般做法是从末状态開始递推:
Homura wants to help her friend Madoka save the world. But because of the plot of the Boss Incubator, she is trapped in a labyrinth called LOOPS.
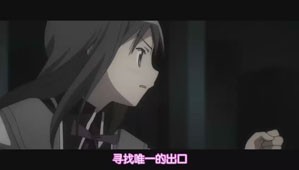
The planform of the LOOPS is a rectangle of R*C grids. There is a portal in each grid except the exit grid. It costs Homura 2 magic power to use a portal once. The portal in a grid G(r, c) will send Homura to the grid below G (grid(r+1, c)), the grid on the right of G (grid(r, c+1)), or even G itself at respective probability (How evil the Boss Incubator is)!
At the beginning Homura is in the top left corner of the LOOPS ((1, 1)), and the exit of the labyrinth is in the bottom right corner ((R, C)). Given the probability of transmissions of each portal, your task is help poor Homura calculate the EXPECT magic power she need to escape from the LOOPS.
The following R lines, each contains C*3 real numbers, at 2 decimal places. Every three numbers make a group. The first, second and third number of the cth group of line r represent the probability of transportation to grid (r, c), grid (r, c+1), grid (r+1, c) of the portal in grid (r, c) respectively. Two groups of numbers are separated by 4 spaces.
It is ensured that the sum of three numbers in each group is 1, and the second numbers of the rightmost groups are 0 (as there are no grids on the right of them) while the third numbers of the downmost groups are 0 (as there are no grids below them).
You may ignore the last three numbers of the input data. They are printed just for looking neat.
The answer is ensured no greater than 1000000.
Terminal at EOF
特殊处理呆在原地概率为1的点。
#include<iostream>
#include<cstdio>
#include<cstring>
#include<algorithm>
#include<limits.h>
typedef long long LL;
using namespace std;
const int maxn=1000+100;
double dp[maxn][maxn];
double p[maxn][maxn][3];
int r,c;int main()
{while(~scanf("%d%d",&r,&c)){for(int i=1;i<=r;i++){for(int j=1;j<=c;j++)scanf("%lf%lf%lf",&p[i][j][0],&p[i][j][1],&p[i][j][2]);}memset(dp,0,sizeof(dp));for(int i=r;i>=1;i--){for(int j=c;j>=1;j--){if(p[i][j][0]==1) continue;else dp[i][j]=(dp[i][j+1]*p[i][j][1]+dp[i+1][j]*p[i][j][2]+2)/(1-p[i][j][0]);}}printf("%.3f\n",dp[1][1]);}return 0;
}
UPC 2225: The number of steps
Description
Mary stands in a strange maze, the maze looks like a triangle(the first layer have one room,the second layer have two rooms,the third layer have three rooms …). Now she stands at the top point(the first layer), and the KEY of this maze is in the lowest layer’s leftmost room. Known that each room can only access to its left room and lower left and lower right rooms .If a room doesn’t have its left room, the probability of going to the lower left room and lower right room are a and b (a + b = 1 ). If a room only has it’s left room, the probability of going to the room is 1. If a room has its lower left, lower right rooms and its left room, the probability of going to each room are c, d, e (c + d + e = 1). Now , Mary wants to know how many steps she needs to reach the KEY. Dear friend, can you tell Mary the expected number of steps required to reach the KEY?
Input
Output
Please calculate the expected number of steps required to reach the KEY room, there are 2 digits after the decimal point.
Sample Input
30.3 0.70.1 0.3 0.60
Sample Output
3.41
#include<iostream>
#include<cstdio>
#include<cstring>
#include<algorithm>
#include<limits.h>
typedef long long LL;
using namespace std;
double a,b,c,d,e;
double dp[50][50];
int main()
{ int n; while(~scanf("%d",&n)&&n) { scanf("%lf%lf%lf%lf%lf",&a,&b,&c,&d,&e); for(int i=1;i<=n;i++) dp[n][i]=i-1; for(int i=n-1;i>=1;i--) { dp[i][1]=dp[i+1][1]*a+dp[i+1][2]*b+1; for(int j=2;j<=i;j++) dp[i][j]=dp[i+1][j]*c+dp[i+1][j+1]*d+dp[i][j-1]*e+1; } printf("%.2f\n",dp[1][1]); } return 0;
}
POJ 2096Description
Two companies, Macrosoft and Microhard are in tight competition. Microhard wants to decrease sales of one Macrosoft program. They hire Ivan to prove that the program in question is disgusting. However, Ivan has a complicated problem. This new program has s subcomponents, and finding bugs of all types in each subcomponent would take too long before the target could be reached. So Ivan and Microhard agreed to use a simpler criteria --- Ivan should find at least one bug in each subsystem and at least one bug of each category.
Macrosoft knows about these plans and it wants to estimate the time that is required for Ivan to call its program disgusting. It's important because the company releases a new version soon, so it can correct its plans and release it quicker. Nobody would be interested in Ivan's opinion about the reliability of the obsolete version.
A bug found in the program can be of any category with equal probability. Similarly, the bug can be found in any given subsystem with equal probability. Any particular bug cannot belong to two different categories or happen simultaneously in two different subsystems. The number of bugs in the program is almost infinite, so the probability of finding a new bug of some category in some subsystem does not reduce after finding any number of bugs of that category in that subsystem.
Find an average time (in days of Ivan's work) required to name the program disgusting.
Input
Output
Sample Input
1 2
Sample Output
3.0000
#include<iostream>
#include<cstdio>
#include<cstring>
#include<algorithm>
#include<limits.h>
typedef long long LL;
using namespace std;
double dp[1100][1000];
double n,s;int main()
{while(~scanf("%lf%lf",&n,&s)){dp[(int)n][(int)s]=0.0;for(int i=n;i>=0;i--){for(int j=s;j>=0;j--){if(i==n&&j==s) continue;dp[i][j] = ( n*s + (n-i)*j*dp[i+1][j] + i*(s-j)*dp[i][j+1] + (n-i)*(s-j)*dp[i+1][j+1] )/( n*s - i*j );}}printf("%.4lf\n",dp[0][0]);}return 0;
}